I was troubled by a mysterious issue where a gap appeared, even though neither margin nor padding was applied to the RelativeLayout inside the Toolbar.
The RelativeLayout is set to match_parent, and the parent element doesn’t have any properties that would create a gap, so why is this happening?
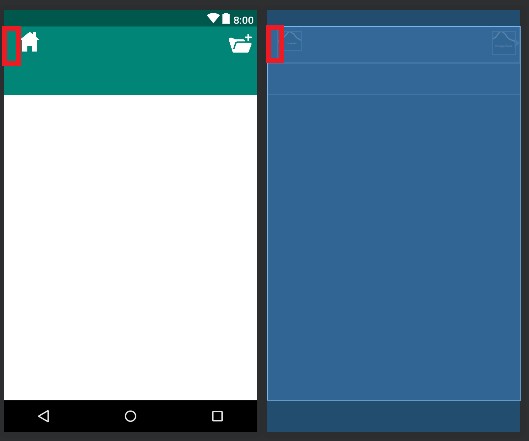
Here’s how the XML looks.
<android.support.v7.widget.Toolbar
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:layout_scrollFlags="scroll|enterAlways" >
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/home_bt"
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_alignParentLeft="true"
android:background="@drawable/icon_home"/>
<ImageView
android:id="@+id/folder_plus"
android:layout_width="35dp"
android:layout_height="35dp"
android:layout_alignParentRight="true"
android:background="@drawable/icon_folder_plus"/>
</RelativeLayout>
</android.support.v7.widget.Toolbar>
There is a mysterious padding on the left side of the HOME button (ImageView @+id/home_bt).
Of course, no margin is specified in the parent element.
Solution
Resolved by adding the contentInsetStart
attribute to the Toolbar and setting the padding to “0”.
<android.support.v7.widget.Toolbar
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
<!-- Add the contentInsetStart element. -->
app:contentInsetStart="0dp"
app:layout_scrollFlags="scroll|enterAlways" >
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/home_bt"
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_alignParentLeft="true"
android:background="@drawable/icon_home"/>
<ImageView
android:id="@+id/folder_plus"
android:layout_width="35dp"
android:layout_height="35dp"
android:layout_alignParentRight="true"
android:background="@drawable/icon_folder_plus"/>
</RelativeLayout>
</android.support.v7.widget.Toolbar>
It seems like a margin is added by default.
I got stuck on this one for a while…